Next.js 11 Images with Static Export
Next.js 11 includes an exciting new feature for dynamically optimising images using the tag. Unfortunately, this new feature does not play nicely with exporting a static version of the Next.js app using yarn next export
by its very design. This is not great for serverless deployments, which often will rely on uploading the static version of web applications to a service like S3 (see my guide of how to that here).
The following provides a simple solution for getting the export working again for your serverless projects. There are more sophisticated solutions that involve custom loaders that allow taking advantage of all the fancy features of Next.js image optimisation, however we are opting here for something simple that will ensure your app still works with a simple static deployment in an S3 bucket.
Here the steps we need to take. All of the following assumes that you are using Next.js 11 for your application.
- Add the next-optimized-images package to your project (along with some additional dependencies it needs)
yarn add next-optimized-images file-loader img-loader url-loader ignore-loader extracted-loader next-compose-plugins
- Import this into the next.config.js of your project
const optimizedImages = require('next-optimized-images');
- Also in your
next.config.js
disable static image rendering as well as initialise thenext-optimized-images
plugin.
const nextConfig = {
images: {
disableStaticImages: true,
},
};
const config = withPlugins(
[
[
optimizedImages,
{
// optimisation disabled by default, to enable check https://github.com/cyrilwanner/next-optimized-images
optimizeImages: false,
},
],
],
nextConfig
);
Also find a complete next.config.js file here.
- If you are using TypeScript, add the image file types as known modules in a
index.d.ts
file in yoursrc/
folder:
declare module '*.svg' {
const content: any;
export default content;
}
declare module '*.png' {
const content: any;
export default content;
}
declare module '*.jpg' {
const content: any;
export default content;
}
declare module '*.jpeg' {
const content: any;
export default content;
}
declare module '*.gif' {
const content: any;
export default content;
}
Now you are ready to run next export
and your images should be included. Small images will be embedded as base64 encoded image directly in your pages and larger images will be copied into a public/static
folder. To see an app rendered in this way see app-next-js.
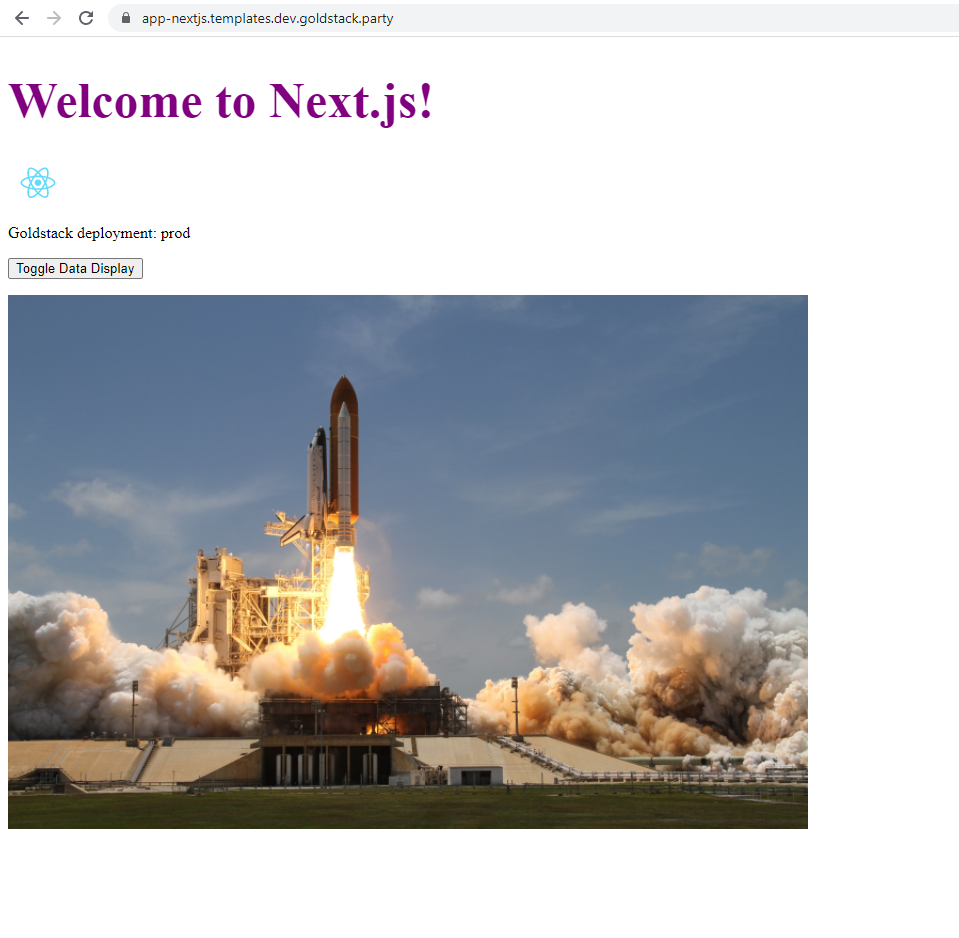
See a completely configured project here: app-next-js.
If you are starting a new project, you can also use the Goldstack Next.js or Goldstack Next.js + Bootstrap templates, which are end-to-end configured for deploying a Next.js application in a serverless way to AWS.